In a C++ function, the variables defined in the function have local scope. They exist as long as they are inside the function and once outside the function they are no longer available. They are initiated when they are defined and go out of scope or destroyed as soon as the function has finished its task.
How do you see this in an actual example. If you run the example, the processing is fast and you do not see the individual steps as to when the variables get defined, when they get initialized and when they go out of scope.
Here a step-by-step process of debugging is described using Visual Studio 2017 Community as in the earlier post.
Let us take this example from an earlier post:
---------------------------
// LocalScopeCNSL.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
using namespace std;
#include
int multiply(int x, int y)
{
return (x * y); // This return value is held in an anonymous object
}
int main()
{
cout << multiply(5, 6);
return 0;
}
-------------
Integer variables x and y are defined as parameters of the function multiply() and therefore they come into existence where they are initially defined. Their return value exists in the main() function and as soon as main() is finished, x and y go out of scope.
Highlight the line:
return (x*y)
Click Debug to open the following:
Debug_0
Pick Toggle Breakpoint and this puts a red dot to the left of the line you highlighted as shown.
With this we arranged to stop the execution at this point and the program will stop here as it begins to execute.
Now go back to Debug and from the menu choose Start Debugging (alternately you can hit F5).
You may get a message like this.
Debug_3
Click Yes.
The program starts executing. You will see the Console comming up and the execution stops at the line you highlighted return(x*y) as shown here:
Debug_4
If you hover your pointed over x or y, you will see that they are defined here.
Debug_5
Now go back to Debug and from the menu click Step Into.
Debug_6
The excution goes forward one step as shown. We will be doing this again and again till the program finishes.
Now hover over cout as shown
Debug_7
Do one more time, Step Into.
The program takes you to where x and y are defined, that is, inside the function multiply().
Debug_8
Take one step forward using Step Into
The program goes to the next step return(x*y) and now x value which was defined is now initiated to 5 as shown. Similarly y is initiated to 6.
Debug_9
Take one more step and you will see that the program has now entered the main() function.
Debug_10
Now move forward one more step and it is now on the line cout. But so far nothing on the Console as shown here:
Debug_11
Step into one more time and the program return to a point just going out of multiply() as shown.
Debug_12
Step Into one more time, the program is in main() again.
The program moves to return 0; and the Console has the value in cout, namely 30 as shown.
Debug_14
If you hover over the variables you will not see their values.
Step Into one more time.
The program is on the verge of finishing as shown.
Debug_15
One more step into and the user program has finished but the processing is not complete and these are being executed as shown:
Hit Continue in the toolbar.
Debug_17
The console closes and the program returns to its original state as shown.
Debug_18
The Program now goes through finishing other details which are internal to the program, part of one step shown here.
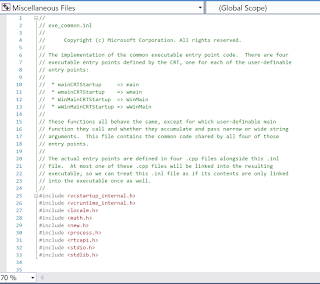
You can click on the red dot to remove the toggle point.
How do you see this in an actual example. If you run the example, the processing is fast and you do not see the individual steps as to when the variables get defined, when they get initialized and when they go out of scope.
Here a step-by-step process of debugging is described using Visual Studio 2017 Community as in the earlier post.
Let us take this example from an earlier post:
---------------------------
// LocalScopeCNSL.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
using namespace std;
#include
int multiply(int x, int y)
{
return (x * y); // This return value is held in an anonymous object
}
int main()
{
cout << multiply(5, 6);
return 0;
}
-------------
Integer variables x and y are defined as parameters of the function multiply() and therefore they come into existence where they are initially defined. Their return value exists in the main() function and as soon as main() is finished, x and y go out of scope.
return (x*y)
Click Debug to open the following:
Debug_0
Pick Toggle Breakpoint and this puts a red dot to the left of the line you highlighted as shown.
With this we arranged to stop the execution at this point and the program will stop here as it begins to execute.
Now go back to Debug and from the menu choose Start Debugging (alternately you can hit F5).
You may get a message like this.
Debug_3
Click Yes.
The program starts executing. You will see the Console comming up and the execution stops at the line you highlighted return(x*y) as shown here:
Debug_4
If you hover your pointed over x or y, you will see that they are defined here.
Debug_5
Now go back to Debug and from the menu click Step Into.
Debug_6
The excution goes forward one step as shown. We will be doing this again and again till the program finishes.
Now hover over cout as shown
Debug_7
Do one more time, Step Into.
The program takes you to where x and y are defined, that is, inside the function multiply().
Debug_8
Take one step forward using Step Into
The program goes to the next step return(x*y) and now x value which was defined is now initiated to 5 as shown. Similarly y is initiated to 6.
Debug_9
Take one more step and you will see that the program has now entered the main() function.
Debug_10
Now move forward one more step and it is now on the line cout. But so far nothing on the Console as shown here:
Debug_11
Step into one more time and the program return to a point just going out of multiply() as shown.
Debug_12
Step Into one more time, the program is in main() again.
The program moves to return 0; and the Console has the value in cout, namely 30 as shown.
Debug_14
If you hover over the variables you will not see their values.
Step Into one more time.
The program is on the verge of finishing as shown.
Debug_15
One more step into and the user program has finished but the processing is not complete and these are being executed as shown:
Hit Continue in the toolbar.
Debug_17
The console closes and the program returns to its original state as shown.
Debug_18
The Program now goes through finishing other details which are internal to the program, part of one step shown here.
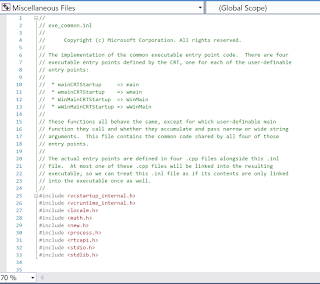
You can click on the red dot to remove the toggle point.
No comments:
Post a Comment